In the previous episode in our Monopoly series we built a flow to handle the effect of a Rule for the active player. In this part, we will integrate it into the main flow and take a closer look at how it works for a Chance or Community Chest squares. These squares are not linked to one single rule but instead involve a stack of cards, each with its own rule.
This time, we will also delve into:
- How do we ensure that the next time a player lands on Chance or Community Chest , the next Rule from the 'deck' is executed?
- How do we shuffle the stack when it is completely empty?
Incorporating the execution of a Rule into the Roll and Move flow
Now we open the SCR – Roll and Move flow, we built in deel 4 again. In this step, we want to process the effect of a Rule linked to a Position after the player arrives at a new Position. In this flow, we see that this (Update Player’s Position) can happen in two different places.
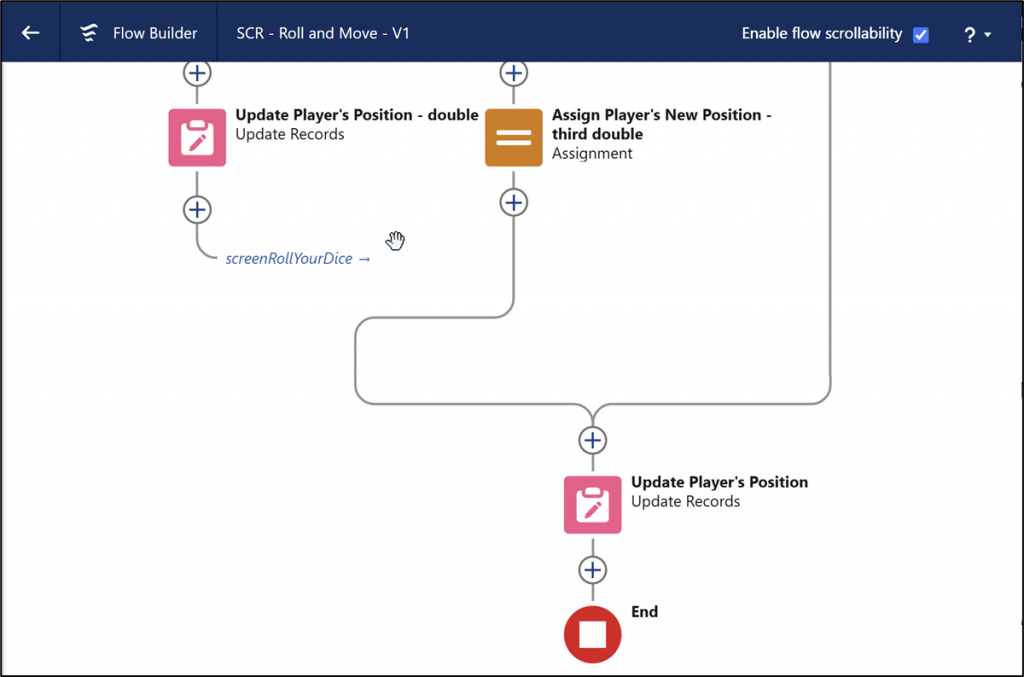
We need a few steps to retrieve the necessary Rule for the SCR – Execute Rule flow - if there is one related to the Position. To avoid building this in two places, we will build a subflow for it. By itself, retrieving a record could be handled in an autolaunched flow, but then we would still need a Decision element in the main flow to determine whether a Rule record was found and whether we should execute the SCR – Execute Rule flow.
We can do this more efficiently by embedding the SCR – Execute Rule flow as a subflow in the flow that checks if there is a Rule connected to the Position. To use a Screenflow as a subflow, the flow you embed it in must also be a Screenflow.
Now that we are introducing this layering, it’s best to start documenting the flow landscape. For this, I’ll use Drawioonce again, which I also used to draw the data model. The following diagram suffices for now. If the landscape becomes more complex, we’ll evaluate whether this setup needs improvement.
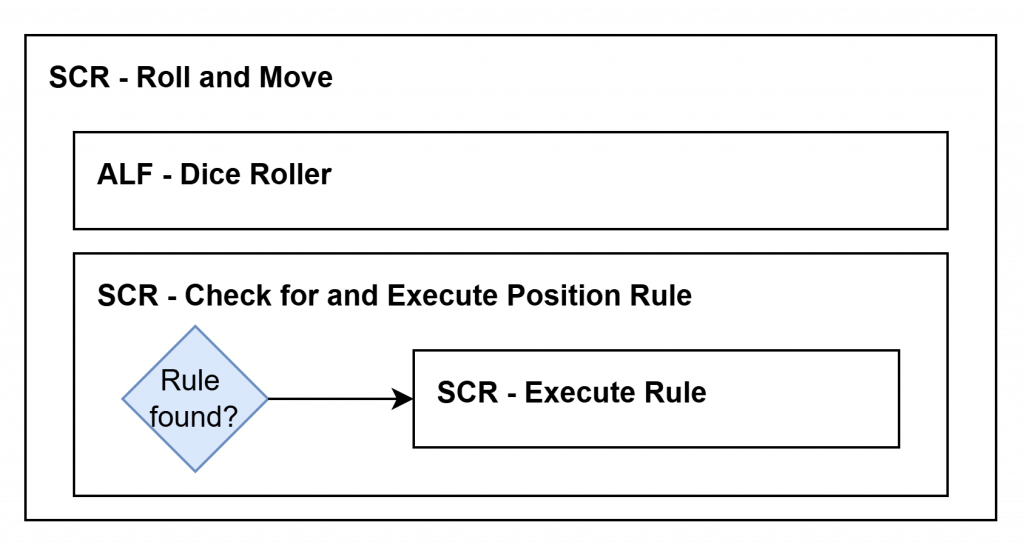
Checking whether a Rule is linked to the Position
Making the SCR – Check for and Execute Position Rule flow receives one input variable from its 'parent' flow: inputPlayer
.
The Player record has already been updated at that point, so the Player's new Position is accessible via the Position lookup. For that Position, we will check for the presence of Position Rules.
Let's first examine the architecture of the decks of Chance and Community Chest cards. In the datamodel episode I explained that we will use a many-to-many relationship between Position and Rule. This allows a single Rule to be associated with multiple Positions, and multiple Rules can also be linked to one single Position.
This way you can link a deck of cards to each Chance square on the board and each Chance card can be connected to each of the Chance squares.
In Salesforce, we create such a many-to-many relationship using a junction object. This is simply a custom object like any other, but the two master-detail relationships and the way we use it makes it junction object.
- Position Rule 1 can link Rule A to Position A.
- Position Rule 2 can link Rule A to Position B.
- Position Rule 3 can link Rule B to Position A.
To arrange the cards in a specific order on the stack, I added an Index
field to the Position Rule object. To pick the top card from the Chance or Community Chest deck, we sort by this Index in our Get Records element. This ensures the player always draws the top card from the stack, the one with the lowest Index number.
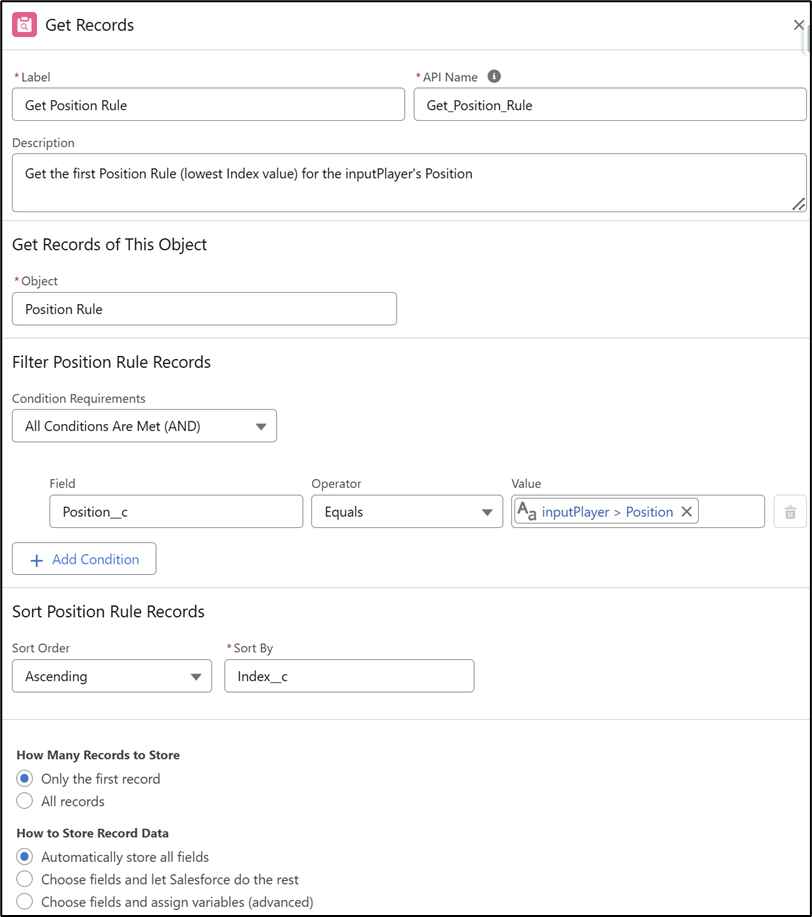
After this Get Records element we use a Decision to check if a Position Rule has been found. After all, there are also Positions that have no Position Rules. In such cases, the rest of this flow does not need to be executed at all.
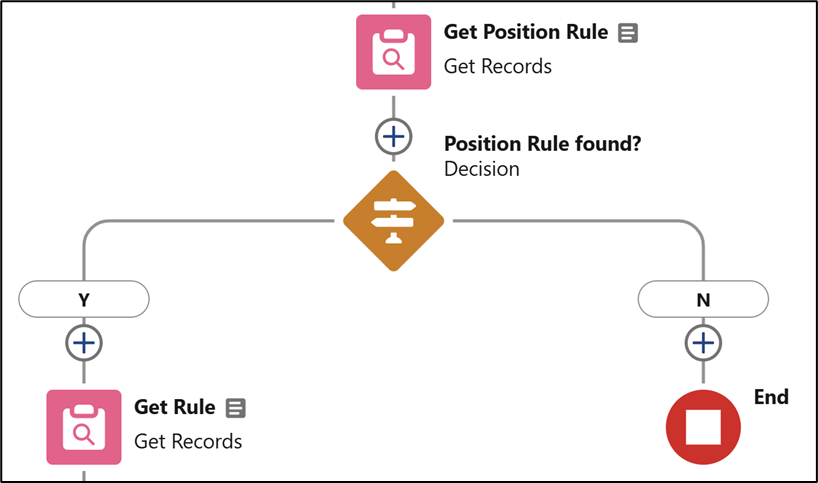
If a Position Rule is found, the next step is to retrieve the Rule itself. We need this just because the SCR – Execute Rule subflow requires a record variable of the Rule object as input and without this query we will not be able to supply that exact record variable to the subflow.
After this Get Records element we will use another null check. hen it confirms that we indeed have a Rule record, we can execute the subflow.
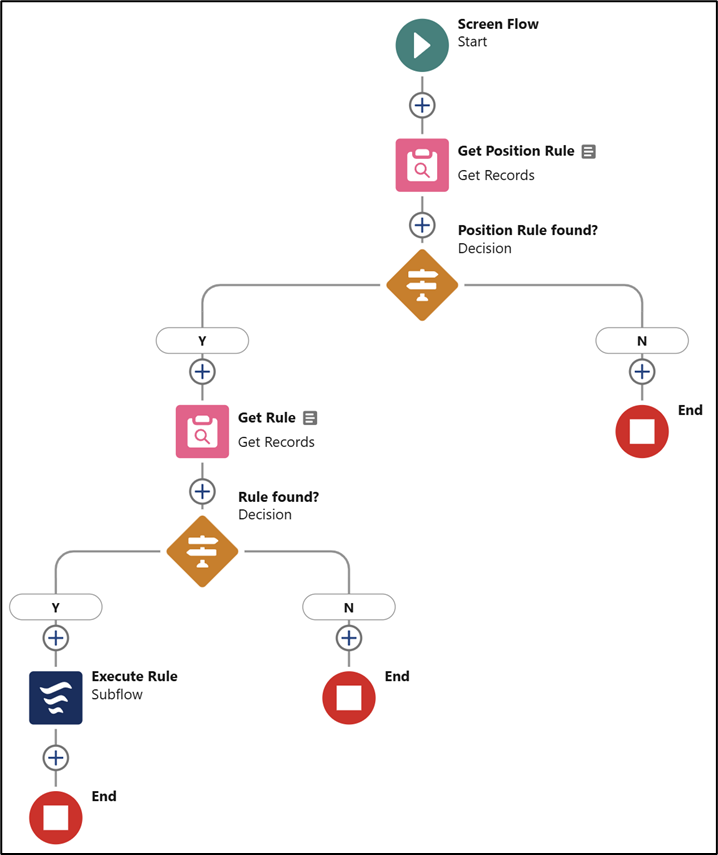
Embedding the subflows
Now we embed this subfow in the SCR – Roll and Move flow in 2 places.
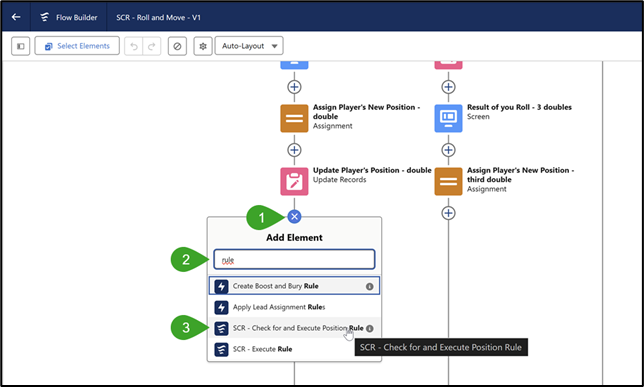
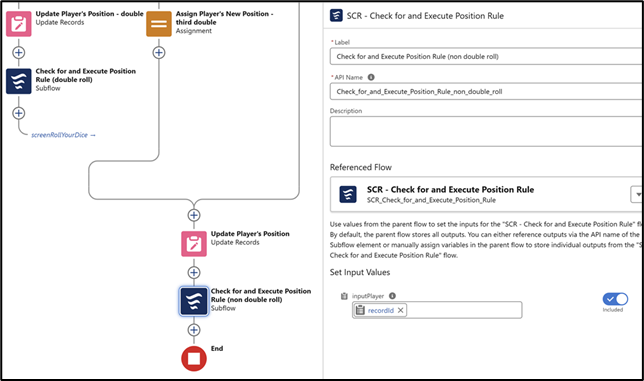
Removing the top Rule of the deck
When a Chance or Community Chest card is used, it is removed from the deck. My first idea was to actually delete all the Position Rules for that particular Rule, but then we would have to recreate them every time the deck is empty. Because for both Chance and Community Chest all new Position Rules would have to be created for 3 Positions for each Rule, this seems more troublesome than keeping the used rules and simply 'setting them aside' for a moment.
So instead we add an update for all Position Rules of the Rule that was just used to the SCR – Check for and Execute Position Rule flow.
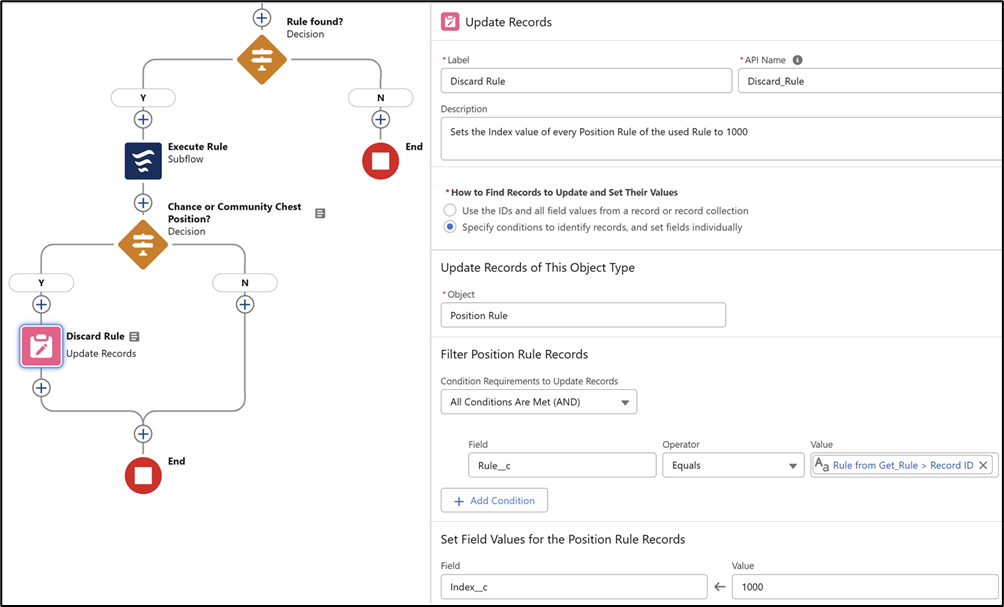
We will also have to modify the Get Position Rule element.
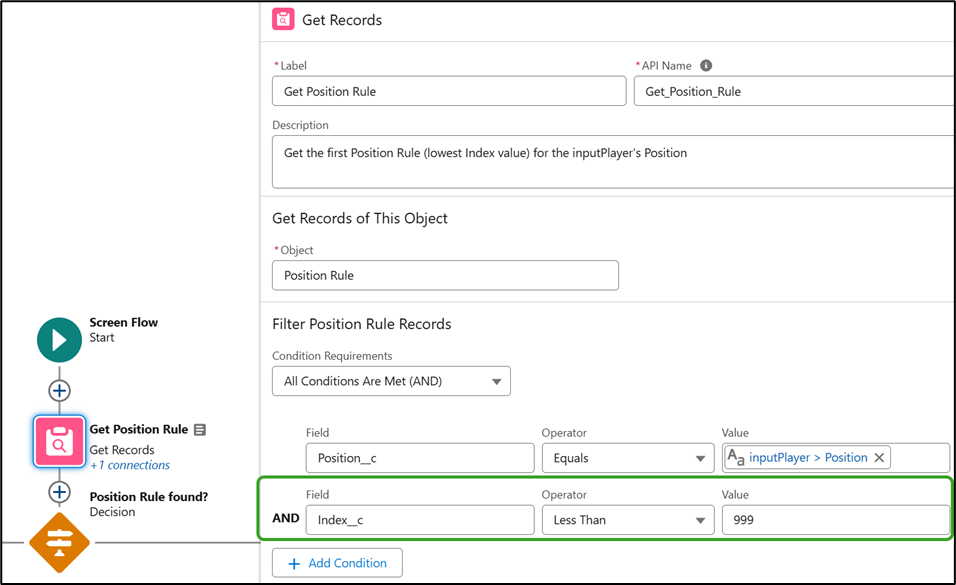
Shuffling the Discard Pile
When the deck is empty, it must be reshuffled. I expect that we cannot build this ourselves with Flow Builder, or we would have to make the flow unnecessarily complex.
I therefore Googled to see if I could find a ready-made Apex Action that does approximately what I want.
Because I can read and write a bit of Apex myself, and since this is not a task for a real client, I feel confident enough to adapt what I found online to fit my needs. Since I am not a developer, I would always have this done by a professional developer when it is needed for an actual client project.
I will also share the code for this Action, which you will use as an element in the Flow. A developer reading this will undoubtedly spot things in the code that could be improved.
cls_deckShuffler
public class cls_deckShuffler {
@InvocableMethod(
label = 'Generate random order'
description = 'Generates a list of integers in random order '
)
public static List<Response> reshuffle( List<Request> requests ) {
List<Response> responsesList = new List<Response>();
for ( Request req : requests ) {
list<Position_Rule__c> inputList = req.inputPositionRulesList;
list<Position_Rule__c> outputlist = new list<Position_Rule__c>();
integer currentIndex = inputList.size();
Position_Rule__c temporaryValue;
integer randomIndex;
// While there remain elements to shuffle...
while (0 != currentIndex) {
// Pick a remaining element...
randomIndex = integer.valueOf(Math.floor(Math.random() * currentIndex));
currentIndex -= 1;
// And swap it with the current element.
temporaryValue = inputList[currentIndex];
inputList[currentIndex] = inputList[randomIndex];
inputList[randomIndex] = temporaryValue;
}
Integer newIndex = 1;
for(Position_Rule__c pr : inputList) {
pr.Index__c = newIndex;
newIndex = newIndex + 1;
outputList.add(pr);
}
Response resp = new Response();
resp.outputPositionRulesList = outputList;
responsesList.add(resp);
} // End requests loop
return responsesList;
} // End converter method
public class Request {
@InvocableVariable(
label = 'Collection of Position Rules to reshuffle'
required = true
)
public list<Position_Rule__c> inputPositionRulesList;
} // End Request Class
public class Response {
@InvocableVariable(
label = 'Collection of reshuffled Position Rules'
)
public list<Position_Rule__c> outputPositionRulesList;
} // End Response Class
} // End cls_DiceRoller
test_deckShuffler
@isTest
private class test_deckShuffler {
@testSetup static void testSetup() {
List<Rule__c> rulesList = new List<Rule__c>();
List<Position_Rule__c> positionRulesList = new List<Position_Rule__c>();
for (integer i = 0; i < 9; i++) {
Rule__c rule = new Rule__c(
Name = String.valueOf(i)
);
rulesList.add(rule);
}
insert rulesList;
Position__c pos = new Position__c(
Name = 'Test Position'
);
insert pos;
for (Rule__c ru : rulesList) {
Position_Rule__c posRule = new Position_Rule__c(
Position__c = pos.Id,
Rule__c = ru.Id,
Index__c = Integer.valueOf(ru.Name)
);
positionRulesList.add(posRule);
}
insert positionRulesList;
}
@isTest
static void unitTest1() {
Test.startTest();
cls_deckShuffler.Request req = new cls_deckShuffler.Request();
req.inputPositionRulesList = [SELECT Id, Name, Index__c, Rule__r.Name FROM Position_Rule__c];
cls_deckShuffler.Request[] requests = new cls_deckShuffler.Request[] {};
requests.add(req);
cls_deckShuffler.Response[] testResults = cls_deckShuffler.reshuffle(requests);
List<Position_Rule__c> results = testResults[0].outputPositionRulesList;
for(Position_Rule__c pr : results) {
system.assertNotEquals(Integer.valueOf(pr.Rule__r.Name), pr.Index__c, 'Position Rule ' + pr.Index__c + ' was not shuffled');
}
Test.stopTest();
}
} // End test_deckShuffler
This Action handles only part of the complete solution we need. The following happens in the Action:
- From the flow, we provide the Action with a record collection of Position Rules as input
- These are rearranged in a new random order
- Then new Index values are set on the records using a loop
- The Action does not commit this change to the database but provides a new record collection with the updated Position Rules as output variabe
In the flow we therefore need to do the following:
- Get all Position Rules where Position is
{!inputPlayer.Position__c}
- Supply this collection as input to the Apex Action
- After the Action, get the Position Rules for every Rule in the deck that are linked to the other Chance Positions on the Board
- Update these so that for each Rule its 3 Position Rules all have the same
Index__c
value, ensuring the deck is stacked in the same order, regardless of which Chance field a player lands on.
That should look as follows
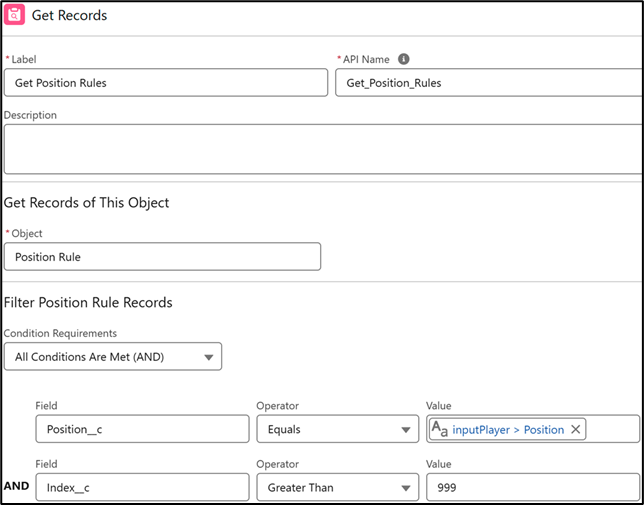
After the Action, I assign its output to a separate updatePositionRules
collection, so I can update all Position Rules at once, including those for the other Chance spaces (or Community Chest), as the Action only modified them for one of the three Positions.
To reorder the Position Rules of all Chance rules linked to the other two Chance squares as well, we need to follow several steps that can be executed using a Flow.
First, we need to populate a text collection with the Id's of all Rules linked to these Position Rules. This collection of Id's will then be used in a subsequent Get records element to retrieve the Position Rules that were not reshuffled yet. Thanks to the Transform element, we don’t need to use a Loop for this.
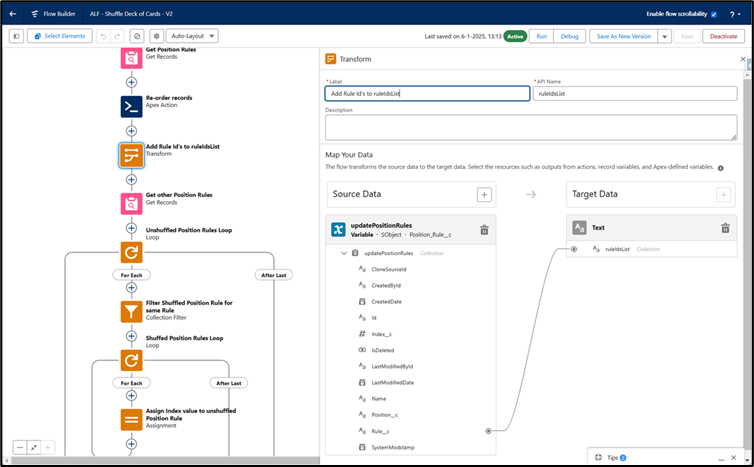
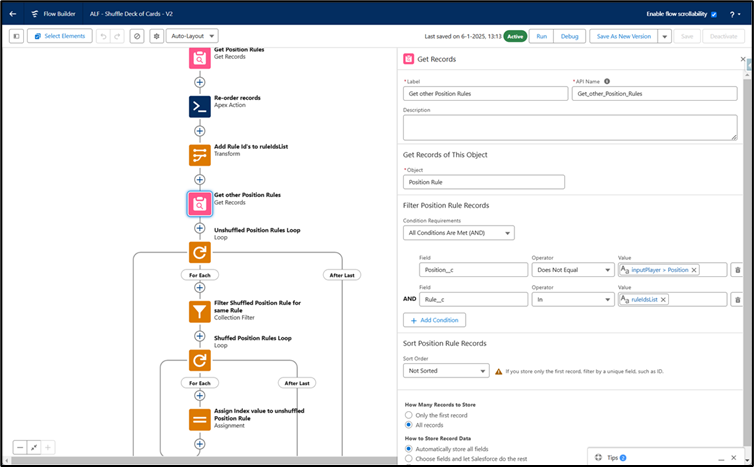
Next, we perform a loop on this Get Other Position Rules collection.
Within the loop, we first filter the updatePositionRules
collection to find the Position Rule corresponding to the same Rule as the current Rule from the collection that has not been reordered yet.
Since a filtered record collection is still a collection, even if it contains only one element, we also need to use a Loop for this.
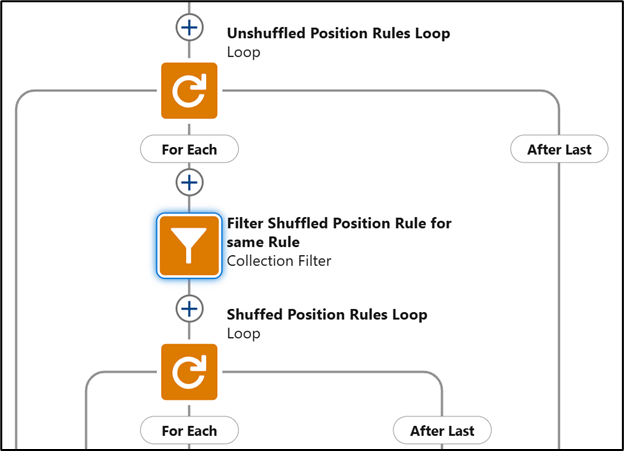
In this inner Loop, we assign the non-reshuffled Position Rule the same Index value as the already updated Position Rule.
Then we exit the inner Loop, but before exiting the outer Loop, we add the now updated Position Rule to the updatePositionRules
collection.
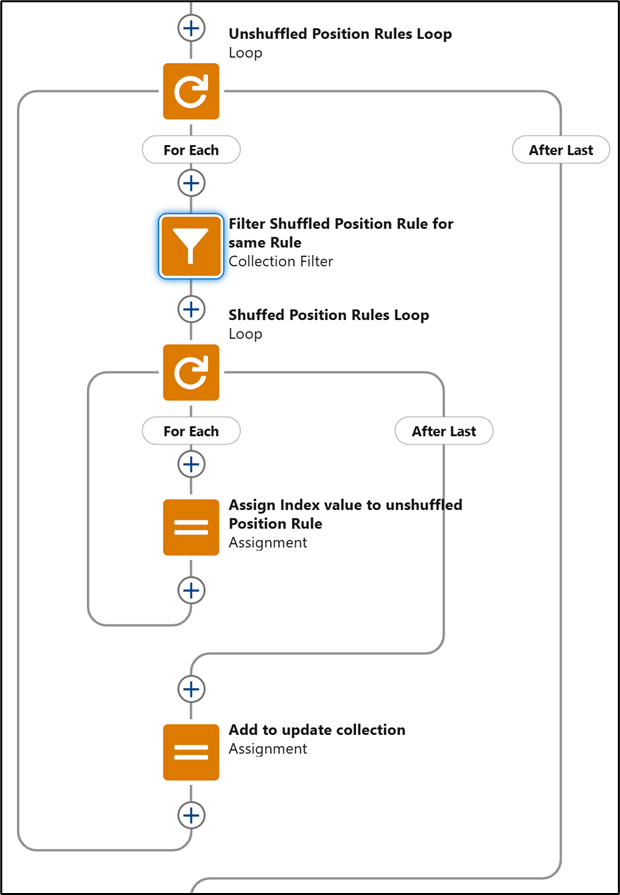
After exiting the outer Loop, we perform the update on all Position Rules for the 16 Rules of this deck, multiplied by the 3 spaces where you need to draw a Rule from that deck.
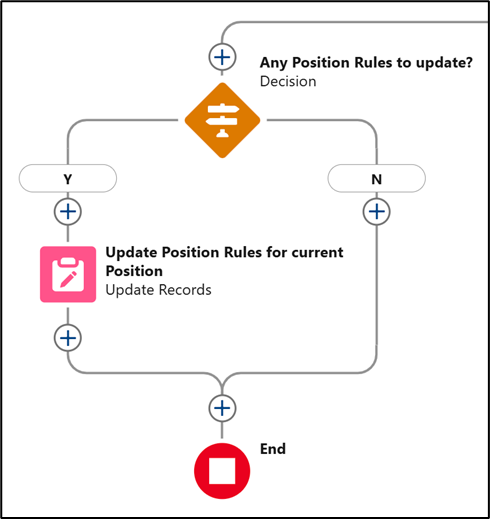
Testing
Now, of course, I want to start testing whether all this works. Some preparation is needed for that.
I will now need to create all the Rules for the Chance and Community Chest cards and link them to the Chance and Community Chest squares using Position Rules.
Since it is supposed to be one deck, I will need to link the same Chance Rule to each Chance square on the board with the same Index value.
Each Chance card is therefore linked to 3 Positions through a Position Rule. Since the deck is always in the same order, the Index__c
for each Position Rule associated with the same Rule must be identical.
The 3 Position Rules for the Chance card Get out of jail free all have Index__c
= 16.
You can download the Excel file below if you’re building along and also want to import the Rules. Once the Rules are imported, we’ll import the Position Rules.
In this file, I have highlighted some rules in yellow. The SCR – Execute Rule flow we built in the previous session cannot yet handle these rules as intended. So, we’ll need to come up with solutions for those later.
Since there is not guarantee that the player will land on Chance or Community Chest after rolling the dice, we will manually place a player on a Chance space for the test and then execute this flow using the Debug button in Flow Builder. I select a Player who is not the Bank as the input variable for my test Flow Interview.
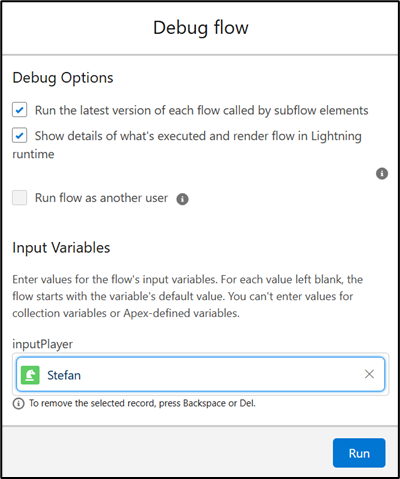
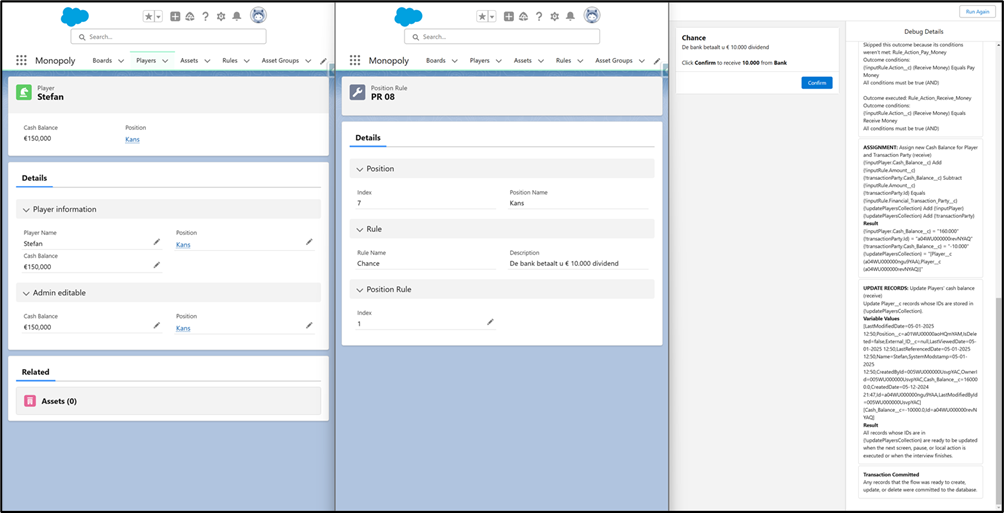
During this test, I discover that an Index of 1,000 is not allowed for Position Rules. Therefore, I need to adjust the flow to update the Index to 999 instead of 1,000.
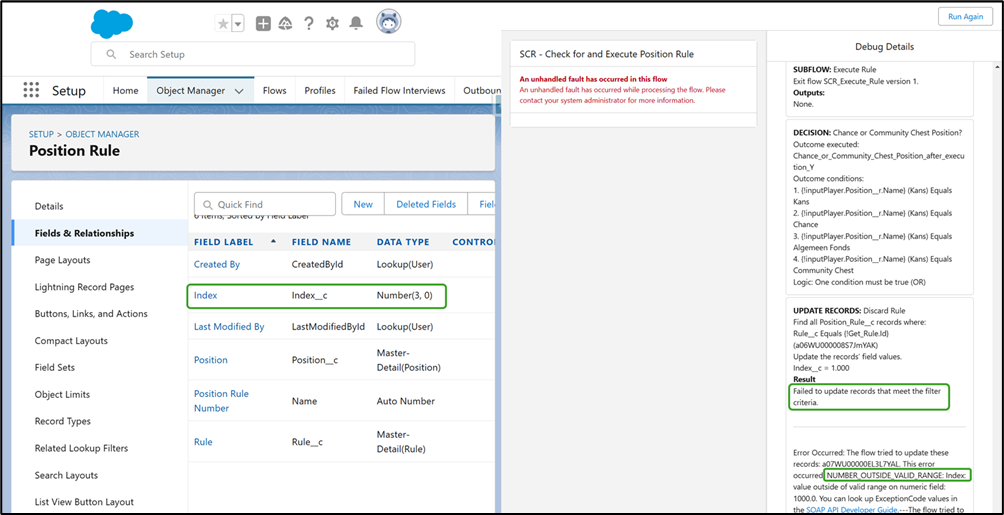
After this adjustment, we run the same Debug test again, and now you can truly see the result of the flow.
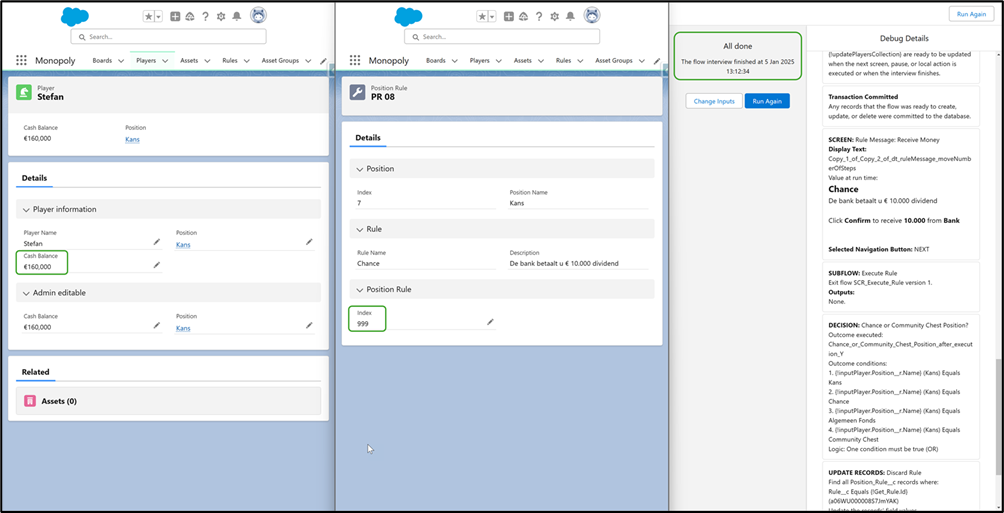
We can also verify via the record page of this Rule that it has indeed been removed from the deck at each Chance square.
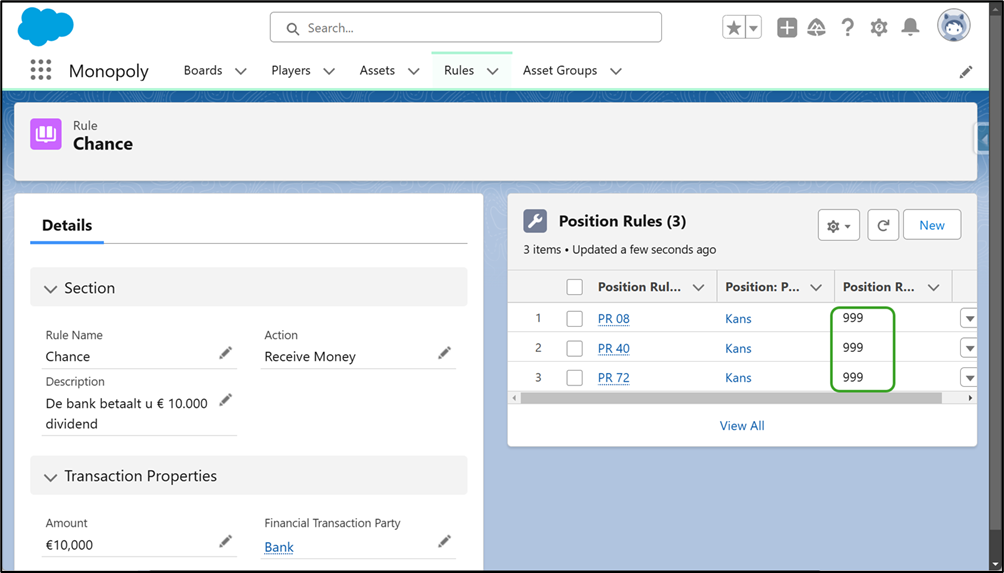
We will also test the flow we built to shuffle the deck of Chance cards.
To start, I will set the Index of each Position Rule for this Position to 999. Then, we will take a screenshot of the page for the Position where our test Player is located.
The we run the ALF – Shuffle Deck of Cards flow with the same Player record we also just inthe previous test.
In the first test run, I received an error because the flow was searching for records with an Index greater than 999 and, therefore, found none. This is related to the adjustment we made based on the previous test. So, we update the filter in the Get Position Rules element from Greater to Greater or Equal.
After this change we encounter no more issues. Below we can see the Position Rules before and after the flow was executed.
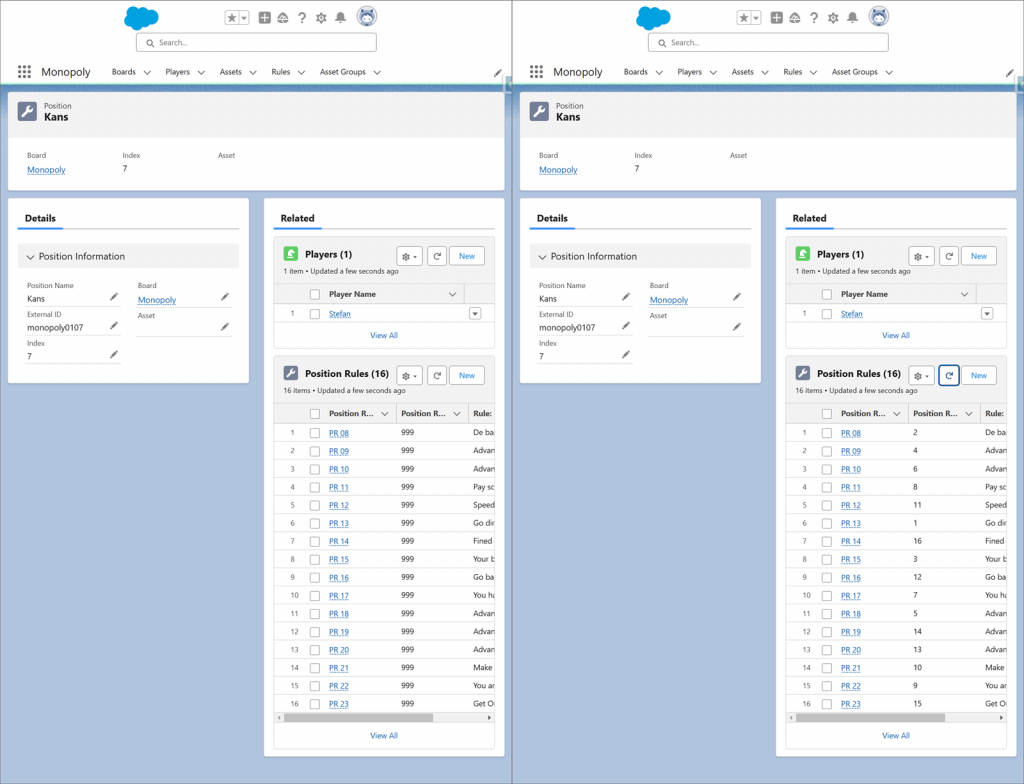
Finally we check if the Position Rules for the same Rule all have the same new Index. And, yes, at all three Chance squares Go directly to jail Do not pass Go Do not collect € 20.000 is on top of the deck.
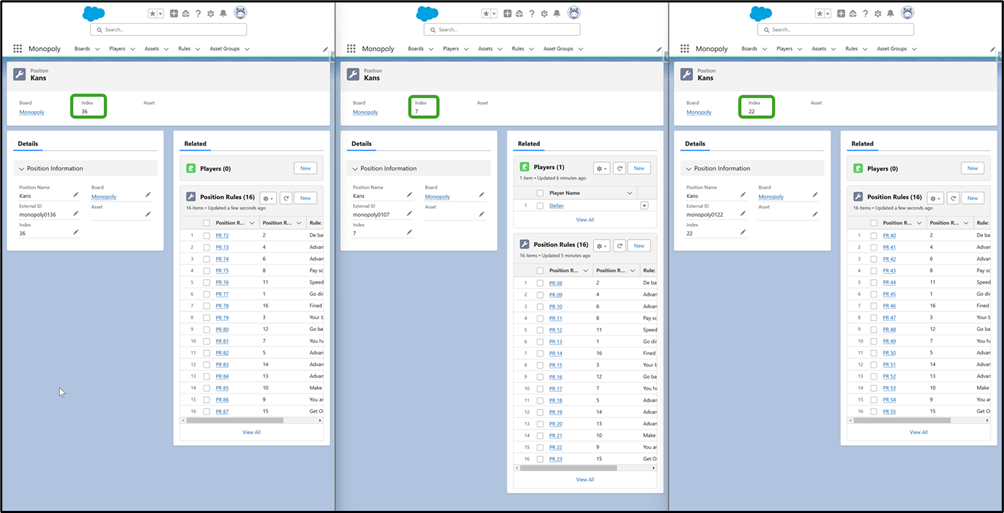
It goes to show that it is important to test when you build flows. When you make a change, immediately test again.
Next time
In the next two episodes we delve into:
- Buying Assets from the bank
- Paying rent
Things we also need to revisit later:
- The Rules that currently cannot yet be executed with the SCR – Execute Rule flow.